Pyboard D-series reference¶
This reference is valid for all PYBD models.
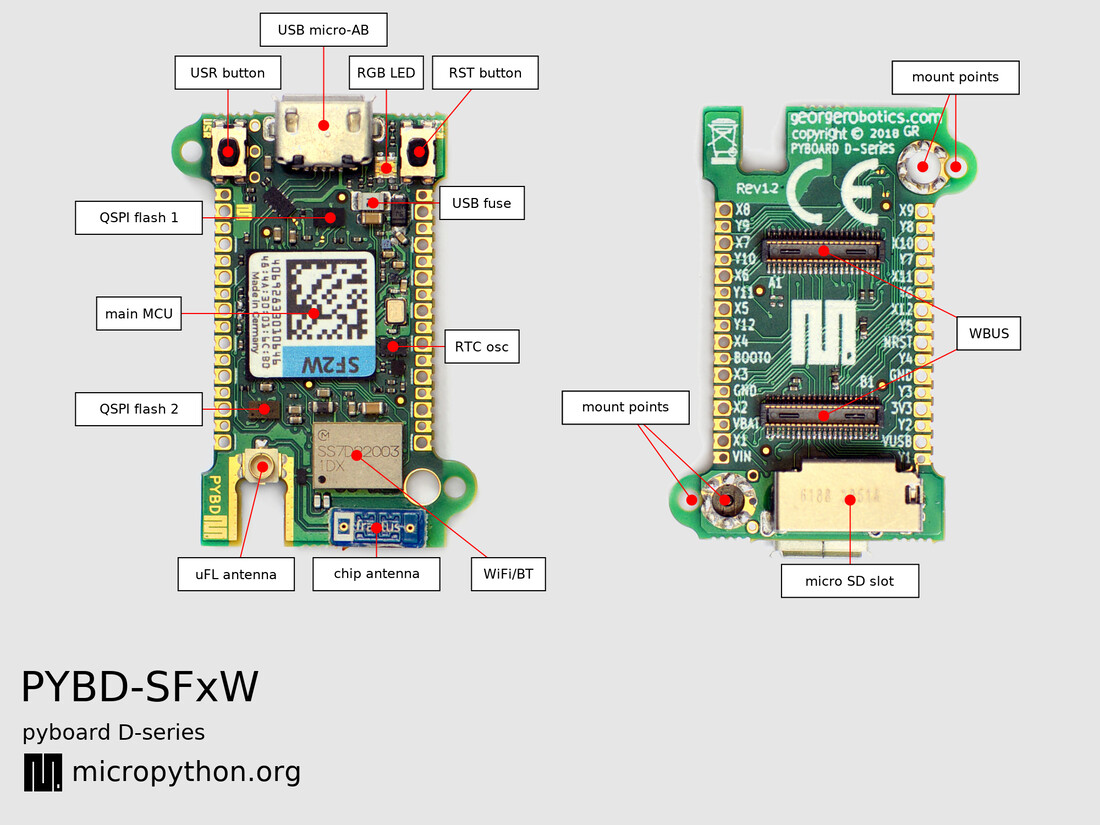
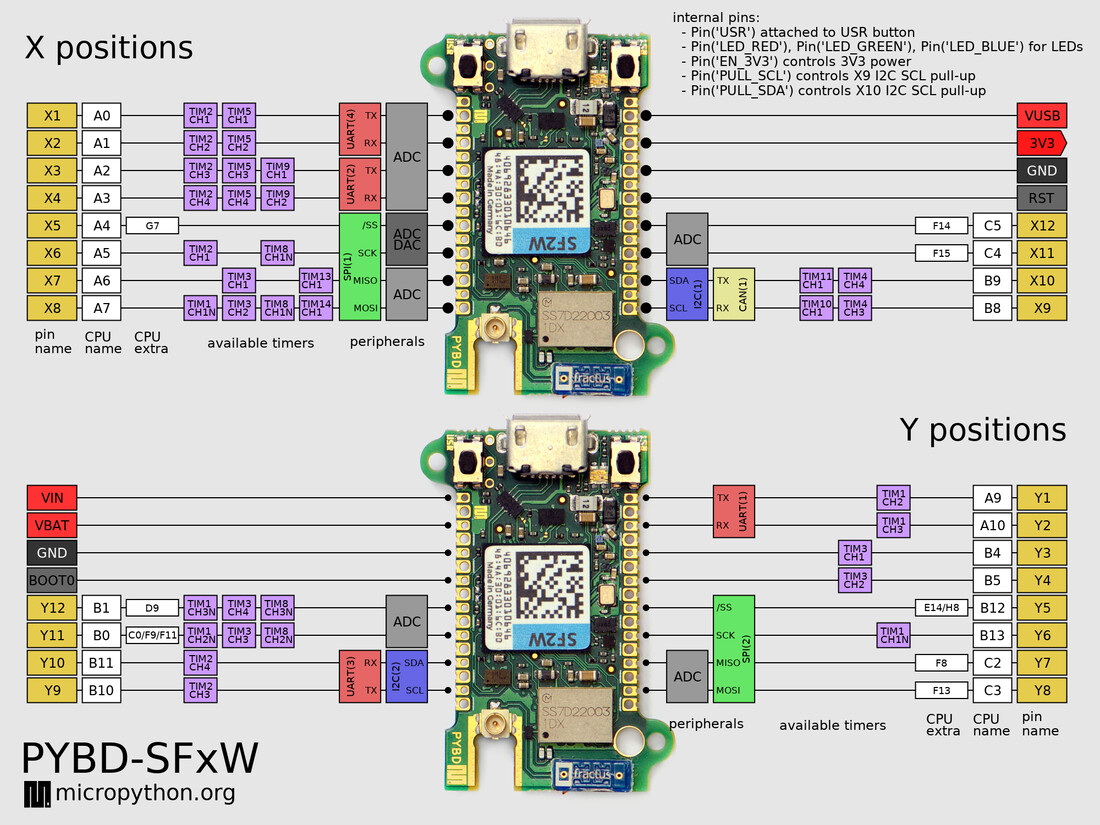
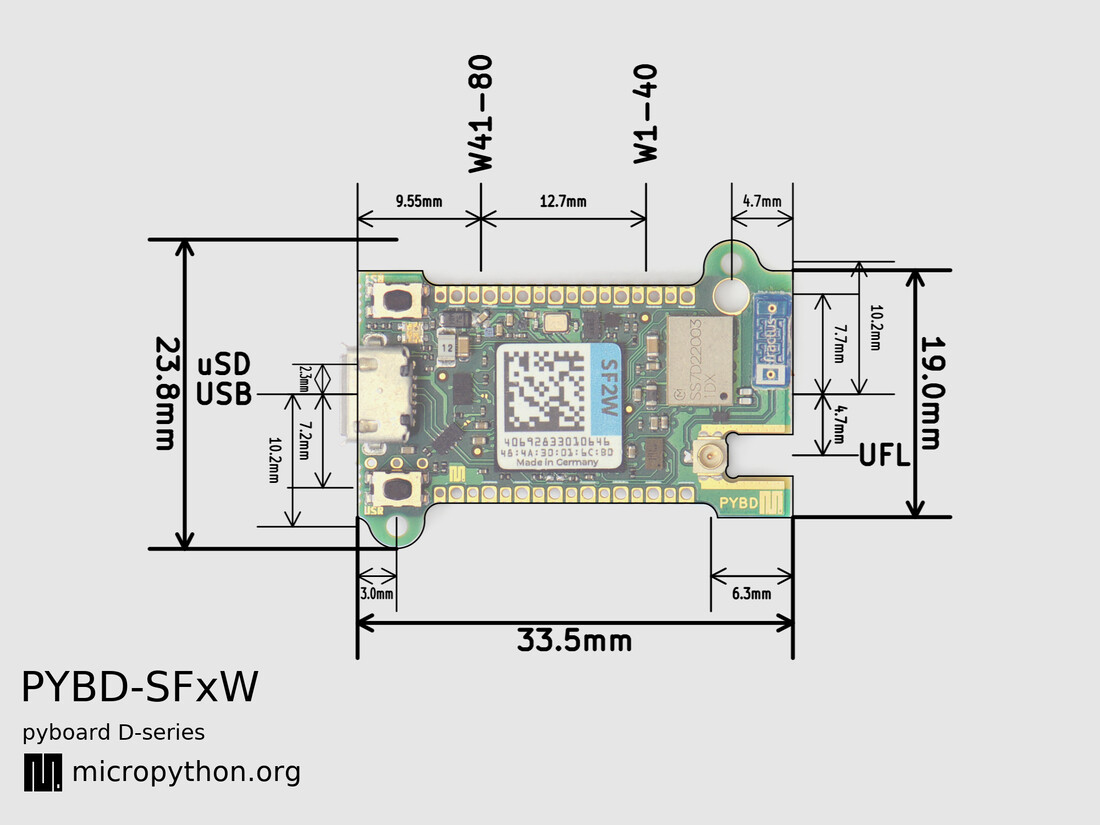
For the schematics see: PYBD_SFxW_schematics.pdf
Power supply¶
Note
Please take note of the following power supply options:
The simplest way is to plug a USB cable into the USB port on the PYBD. That will power the PYBD and allow communication with the PC.
You can power the PYBD via the VUSB port (see X-positions). The voltage on this port must be between 4.8V and 5.2V. This port contains a protective diode and a 1A fast/0.22Ohm fuse.
You can power the PYBD via the VIN port (see Y-positions). The voltage on this port must be between 3.2V and 4.8V.
The 3V3 pin is output only and should not be used to power the board. It can be used as a regulated 3.3V output supply, maximum 300mA total output current including power consumed by the SD card or eMMC.
General recommendations for powering PYBD from a custom power source:
For best performance provide 3.4V on VIN (3.3V is also ok but 3.4V will benefit from LDO filtering). If there is a chance that the USB port (or VUSB) will provide power as well as VIN, then VIN should be protected with a diode (eg Schottky diode or a FET reverse protector).
If supply voltage is 5V then either provide it on VUSB (only available on the X-position through-holes) or put a diode inline and feed into VIN (diode should drop voltage to 4.8V or below).
A summary of the power ports is provided in the table below. For full details please consult the schematics.
Port |
Use |
Voltage |
Notes |
---|---|---|---|
USB conn |
power and comms |
5V |
connect to PC or USB power brick |
VUSB |
power in |
4.8V-5.2V |
1A fast/0.22Ohm fuse and protective diode |
VIN |
power in |
3.2V-4.8V |
also on WBUS |
VBAT |
backup battery |
1.65V-3.6V |
also on WBUS |
3V3 |
output only |
3.3V |
user switchable LDO max 300mA total |
Warning: If the USB host port which powers PYBD is capable of delivering more than 1A, a shortcut on VIN would trip the built-in fuse on the PYBD.
Note: VIN may also be used as a non switchable power supply output as long as total current is below 1A.
Power control¶
The 3V3 output port is connected internally to an LDO which is switchable on
and off via the Pin('EN_3V3')
pin. It is turned on by default during the boot
sequence.
The CPU frequency can be set to any multiple of 2MHz between 48MHz and 216MHz, via
machine.freq(<freq>)
. By default the SF2 model runs at 120MHz and the SF6 model
at 144MHz in order to conserve electricity. It is possible to go below 48MHz but then
the WiFi cannot be used.
Filesystem¶
The PYBD has an internal 2MiB filesystem which is FAT formatted and available to Python
at the path '/flash'
. The underlying block device for this filesystem is available
via the pyb.Flash()
object.
USB ports¶
There are two USB interfaces: FS and HS. The USB HS port is the main one and is
available on the PYBD itself. The FS port is made available on the WBUS-DIP28
adapter. By default the USB port to use will be auto-detected based on the presence
of a cable plugged in. It can be selected manually, eg in boot.py
:
import pyb
pyb.usb_mode('VCP+MSC', port=-1) # default, auto-detect port
pyb.usb_mode('VCP+MSC', port=0) # select FS port
pyb.usb_mode('VCP+MSC', port=1) # select HS port
pyb.usb_mode()
can be called at anytime, from any script, but is usually put
in boot.py
to configure the USB as early as possible in the boot sequence.
You can use pyb.usb_mode(None)
to completely disable and turn off the USB
peripheral (this saves power).
When MSC mode is enabled the connected PC will be able to see the filesystem of
the PYBD. By default this is the internal 2MiB flash, unless an SD card is inserted
in which case it is the SD card. This can be selected manually via the msc
keyword argument which should be a tuple or list of one element, for example:
pyb.usb_mode('VCP+MSC', msc=(pyb.Flash(),)) # expose internal flash to the PC
pyb.usb_mode('VCP+MSC', msc=(pyb.SDCard(),)) # expose SD card to the PC
pyb.usb_mode('VCP+MSC', msc=(pyb.MMCard(),)) # expose MMC to the PC
WiFi control¶
In your boot.py
file you should configure the country for the allowed WiFi
channels in your region:
import pyb
pyb.country('US') # 2-char code, eg: US, GB, DE, NL, FR, AU, CA
To use the WiFi station interface:
import network
wl = network.WLAN()
wl.active(1) # bring up the interface
wl.config('mac') # get the MAC address
wl.config(antenna=0) # select antenna, 0=chip, 1=external
wl.scan() # scan for access points, returning a list
wl.connect('ssid', 'password') # connect to an access point
wl.isconnected() # check if connected to an access point
wl.disconnect() # disconnect from an access point
To use the WiFi access-point interface:
import network
wl_ap = network.WLAN(1)
wl_ap.config(essid='PYBD') # set AP SSID
wl_ap.config(password='pybd0123') # set AP password
wl_ap.config(channel=6) # set AP channel
wl_ap.active(1) # enable the AP
wl_ap.status('stations') # get a list of connection stations
wl_ap.active(0) # shut down the AP
See network
for more details.
Internal LEDs¶
See pyb.LED.
from pyb import LED
led = LED(1) # 1=red, 2=green, 3=blue
led.toggle()
led.on()
led.off()
Internal USR button¶
See pyb.Switch.
from pyb import Switch
sw = Switch()
sw.value() # returns True or False
sw.callback(lambda: pyb.LED(1).toggle())
Pins and GPIO¶
All pins are available via their WBUS name, eg Pin('W19')
.
Some pins also have other names for convenience, such as Pin('X1')
.
See pinouts for a full list of accessible pin names.
See pyb.Pin for details of pin use.
from machine import Pin
x1 = Pin('X1', Pin.OUT)
x1.value(1) # set pin digital high
x2 = Pin('X2', Pin.IN, Pin.PULL_UP)
print(x2.value()) # read digital value
I2C buses¶
The I2C bus on the X position, pins X9 and X10, has two independent user selectable
pull-up resistors on SCL and SDA, of 5.6kOhm each. They are connected on their
high side to Pin('PULL_SCL')
and Pin('PULL_SDA')
and are disabled by default.
To enable them use:
from machine import Pin
Pin('PULL_SCL', Pin.OUT, value=1) # enable 5.6kOhm X9/SCL pull-up
Pin('PULL_SDA', Pin.OUT, value=1) # enable 5.6kOhm X10/SDA pull-up
RTC (real time clock)¶
The internal RTC is driven by a high precision, pre-calibrated oscillator. See pyb.RTC for details of RTC use.
from pyb import RTC
rtc = RTC()
rtc.datetime((2017, 8, 23, 1, 12, 48, 0, 0)) # set a specific date and time
rtc.datetime() # get date and time
SD card¶
The board has a built-in micro SD card slot. If an SD card is inserted, by
default it will not be automatically mount in the board’s filesystem but it will
be exposed as a mass storage device if USB is used. To automatically mount the
SD card if it is inserted, put the following in your boot.py
:
import sys, os, pyb
if pyb.SDCard().present():
os.mount(pyb.SDCard(), '/sd')
sys.path[1:1] = ['/sd', '/sd/lib']
The above code will also put the SD card’s filesystem in the path list so Python scripts can be imported from the SD card. You can remove or change this as desired.
Differences to the original PYBv1.x¶
The main differences between PYBD and the original PYBv1.x pyboard are:
If inserted, the SD card is not automatically mounted on PYBD.
The last (8th) element of
pyb.RTC().datetime()
is microseconds and counts up on PYBD.